I am building a web app using React, Django and AWS. I have a Rest API on API Gateway that is giving a 403 "MissingAuthenticationTokenException" that seems to be specifically on OPTIONS requests. I am quite confused as I haven't set any authentication on the API and even have included an API key to try and fix it.
This is what the error looks like on the console:
Console Error: 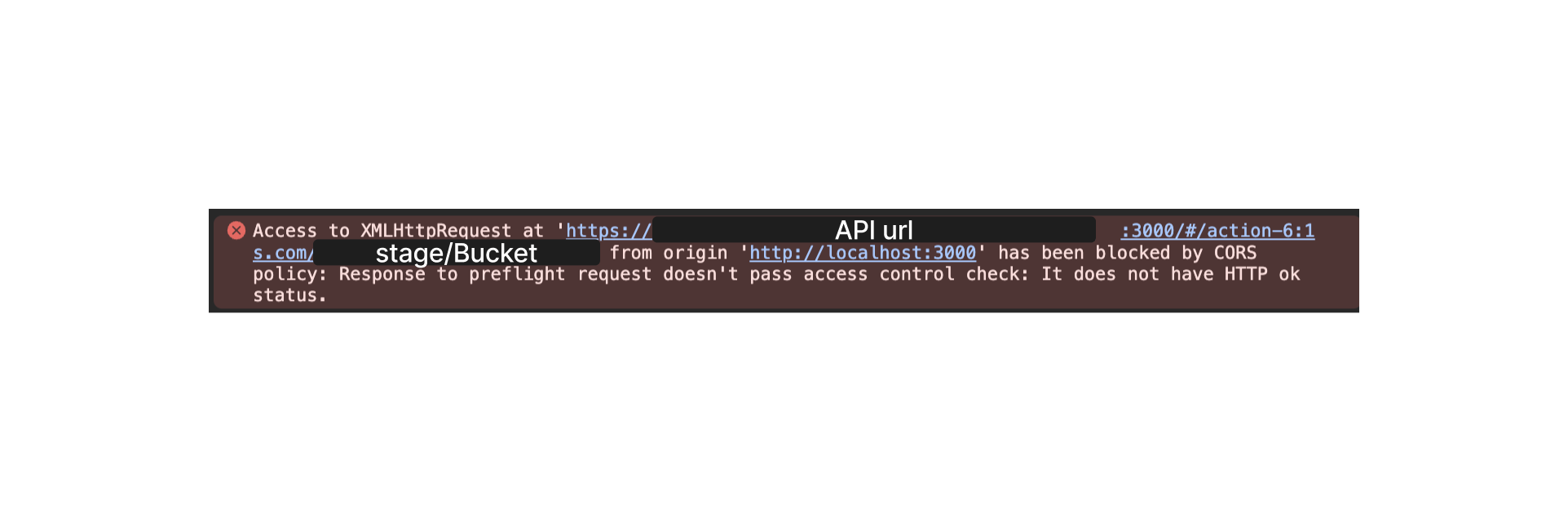
And when I looked at the network I see that it is caused by a 403 error:
Preflight Error: 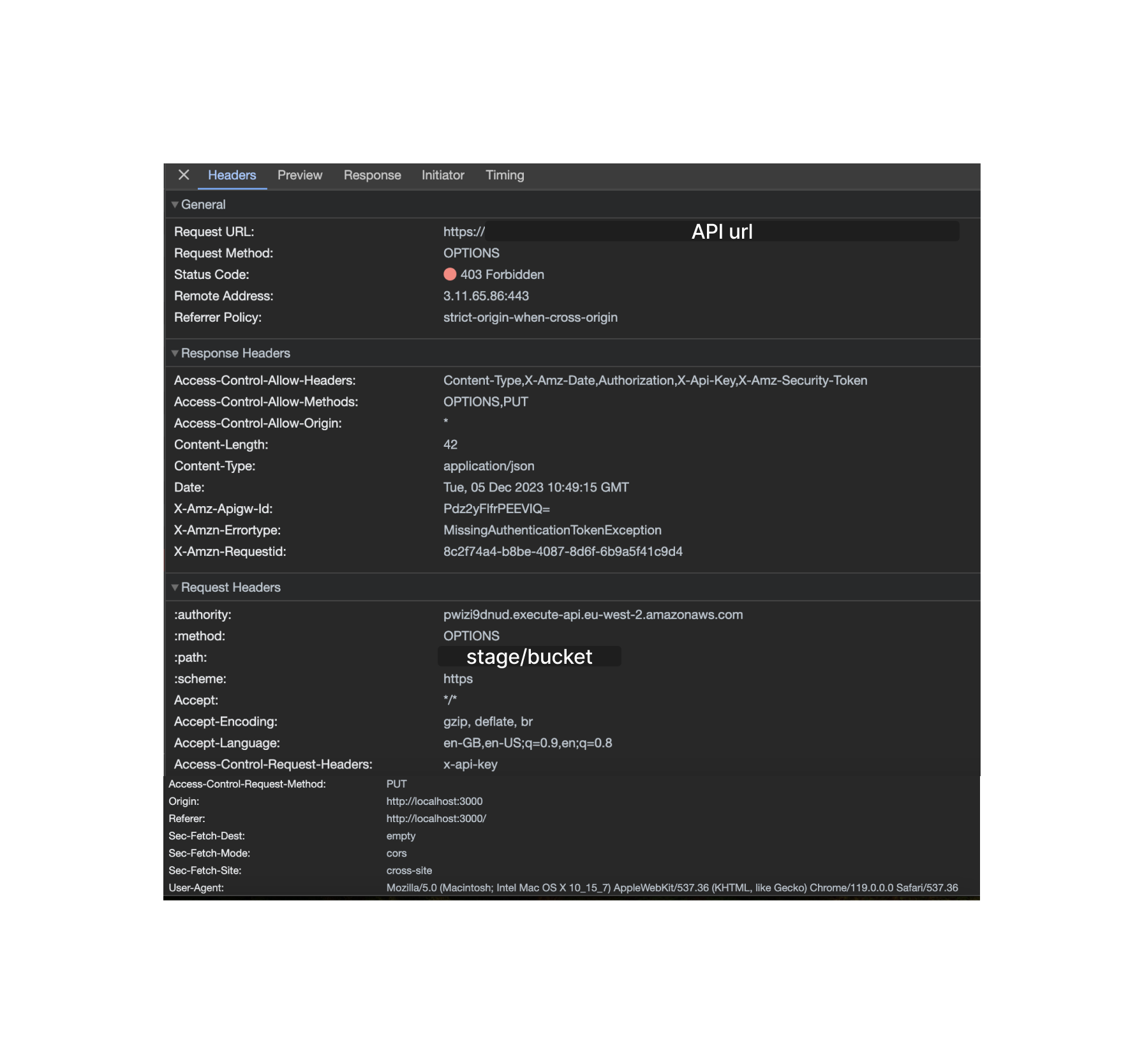
Cors Error: 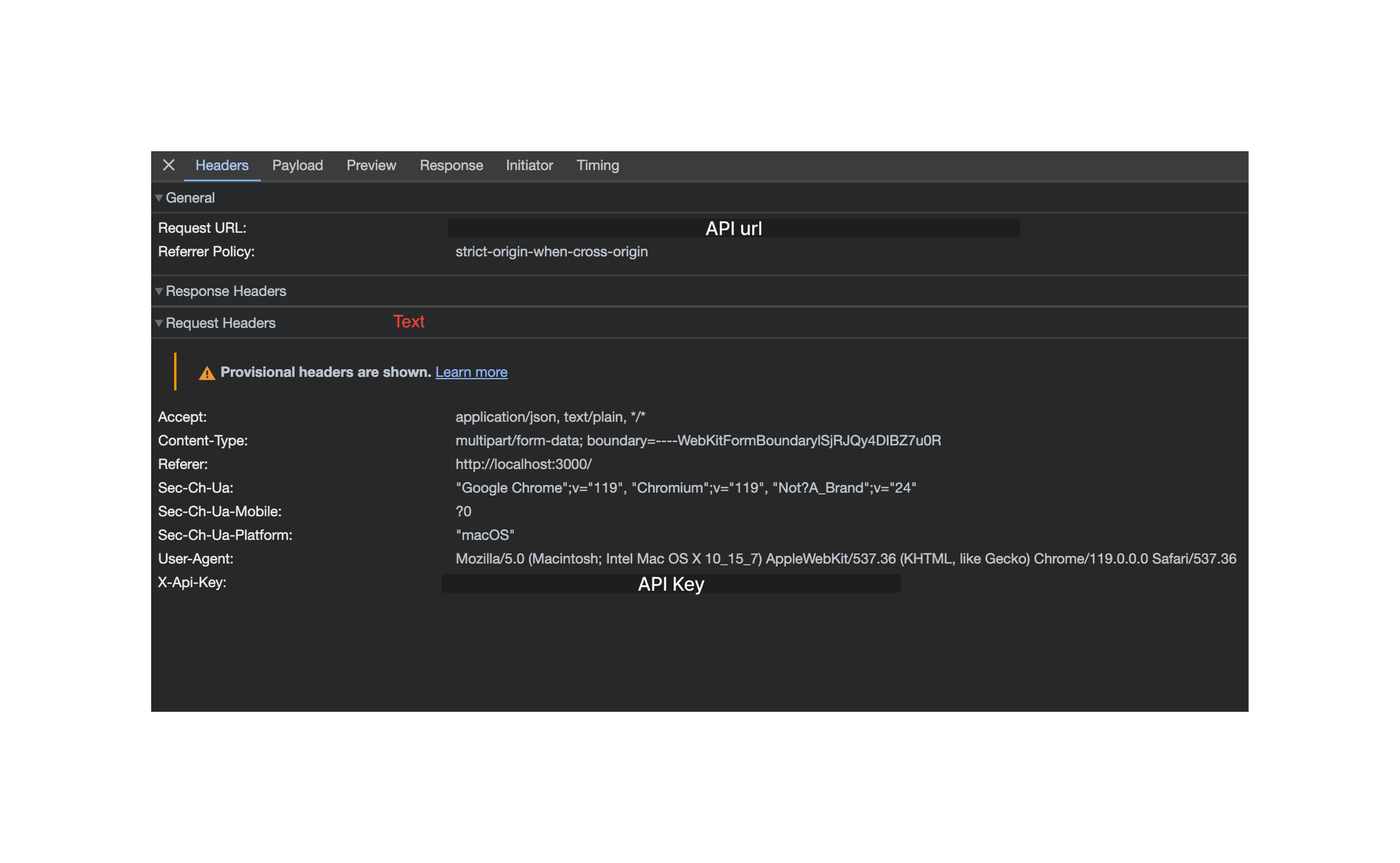
Network Error: 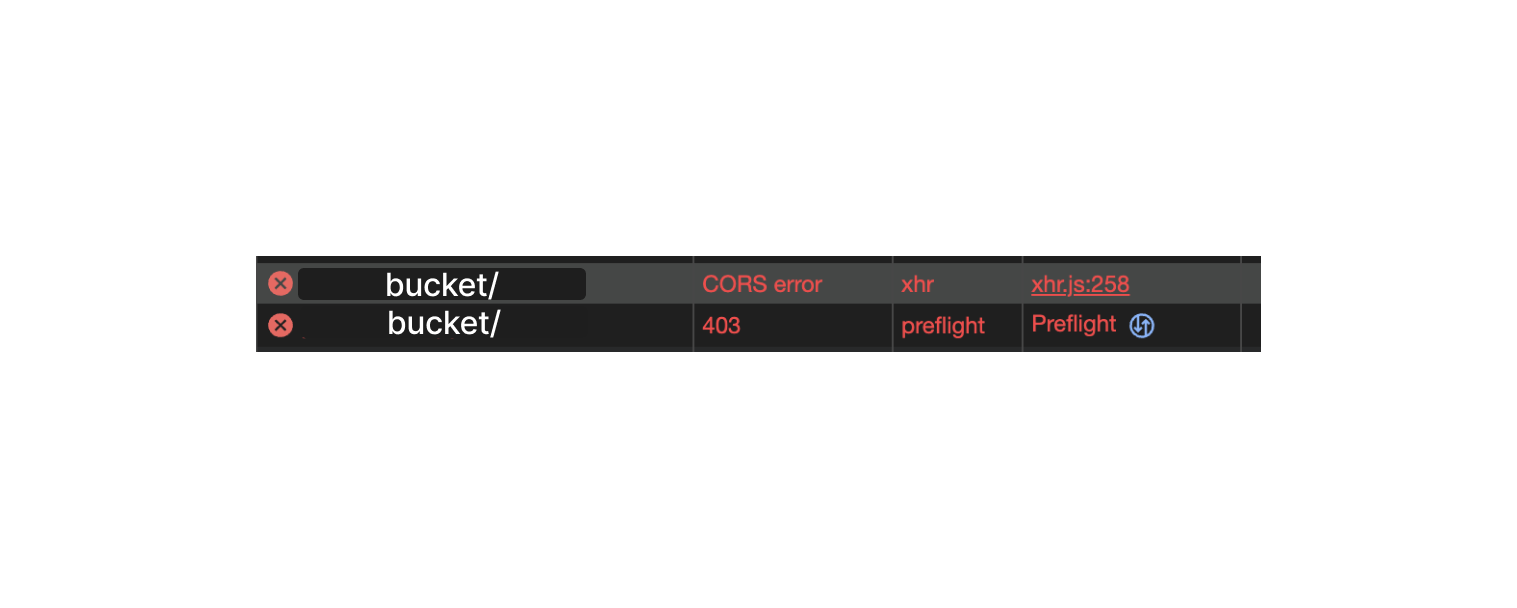
On my react front-end I call the API on a button press:
const formData = new FormData();
formData.append("image", selectedFile);
try {
await axios({
method: "put",
url: "https://my-api.execute-api.eu-west-2.amazonaws.com/dev/photos/",
data: formData,
headers: {
"Content-Type": "multipart/form-data",
"x-api-key": "abc123"
}
});
} catch (error) {
console.error(error);
}
And on my Django views.py it looks like this:
def upload_image(request, bucket, filename):
if request.method == 'OPTIONS':
# Respond to preflight request with a 200 OK
response = Response({'message': 'Preflight request successful'})
response['Access-Control-Allow-Origin'] = '*' # Update with your specific origin(s)
response['Access-Control-Allow-Methods'] = 'PUT, OPTIONS'
response['Access-Control-Allow-Headers'] = 'Content-Type, X-Amz-Date, Authorization, X-Api-Key, X-Amz-Security-Token'
response.statusCode = 200 # Set the status code to 200 for OPTIONS requests
return response
try:
# Your existing code for handling image upload
file = request.data['image']
# Use boto3 to upload the image to AWS S3
s3 = boto3.client(
's3',
aws_access_key_id=os.getenv('AWS_ACCESS_KEY_ID'),
aws_secret_access_key=os.getenv('AWS_SECRET_ACCESS_KEY')
)
s3.upload_fileobj(file.file, bucket, filename)
# Return success response
return Response({'message': 'Image uploaded successfully'}, status=status.HTTP_201_CREATED)
except Exception as e:
# Print the exception for debugging
print(f"Error: {str(e)}")
# Return a more informative error response
return Response({'error': 'Error uploading image. Please check the server logs for more details.'}, status=status.HTTP_400_BAD_REQUEST)
To try and fix the error I have tried to put in the API key into the code which doesn't seem to fix anything. I have tried to make a if statement for my upload_image to fix this, I have enabled cors and followed a lot of advice on how to fix it online but none of it seems to work.
What could be causing the OPTIONS requests to fail the preflight CORS check in API Gateway when I have enabled CORS on all methods?