Hello guys, how are you I am a beginner in AWS and I wanna know can I use plain JavaScript to store values and manipulate them using DynamoDB.
const { DynamoDBClient } = require('@aws-sdk/client-dynamodb');
const { DynamoDBDocument } = require('@aws-sdk/lib-dynamodb');
// Create service client module using ES6 syntax.
//import { DynamoDBClient } from "@aws-sdk/client-dynamodb";
// Set the AWS Region.
const REGION = "ap-south-1"; //e.g. "us-east-1"
// Create an Amazon DynamoDB service client object.
const ddbClient = new DynamoDBClient({ region: REGION });
// Import required AWS SDK clients and commands for Node.js
import { CreateTableCommand } from "@aws-sdk/client-dynamodb";
// Set the parameters
const params3 = {
AttributeDefinitions: [
{
AttributeName: "Season", //ATTRIBUTE_NAME_1
AttributeType: "N", //ATTRIBUTE_TYPE
},
{
AttributeName: "Episode", //ATTRIBUTE_NAME_2
AttributeType: "N", //ATTRIBUTE_TYPE
},
],
KeySchema: [
{
AttributeName: "Season", //ATTRIBUTE_NAME_1
KeyType: "HASH",
},
{
AttributeName: "Episode", //ATTRIBUTE_NAME_2
KeyType: "RANGE",
},
],
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1,
},
TableName: "TEST_TABLE", //TABLE_NAME
StreamSpecification: {
StreamEnabled: false,
},
};
const run = async () => {
try {
const data = await ddbClient.send(new CreateTableCommand(params3));
console.log("Table Created", data);
return data;
} catch (err) {
console.log("Error", err);
}
};
run();
and I am facing this error-
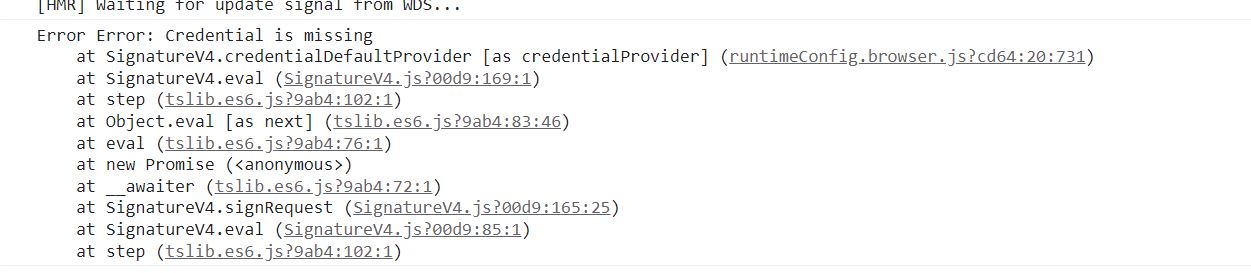
above is a code and output image in which I am facing credentials error. Please someone guide me where I am doing wrong. Thanks for your time and guidance.
Like I am using JavaScript in my local machine can you tell me which is the exactly process for passing credentials?
Run
aws configure
from the CLI. It will set up credentials locally and is the preferred method.https://docs.aws.amazon.com/cli/latest/userguide/cli-configure-quickstart.html
Otherwise you can add the credentials to your DynamoDB Client directly, but it is strongly discouraged to do so.